Password authentication is a fundamental aspect of security in any application. It ensures that only authorized users can access certain functionalities or data. In this blog post, we will delve into the basics of password authentication using Python. We will cover the necessary Python code, explain how it works, and provide tips for enhancing security. By the end, you’ll have a solid understanding of how to implement password authentication in your Python projects.
Introduction to Password Authentication
Password authentication is a security mechanism used to verify the identity of a user by requiring them to provide a password. The password is compared to a stored value (usually hashed and salted for security), and if they match, the user is granted access. This process is crucial in protecting sensitive information and preventing unauthorized access.
Implementing Basic Password Authentication in Python
Let’s start with a basic implementation of password authentication using Python. We will use the getpass
module to securely handle password input.
The Code
Here’s a simple example to illustrate how password authentication can be implemented:
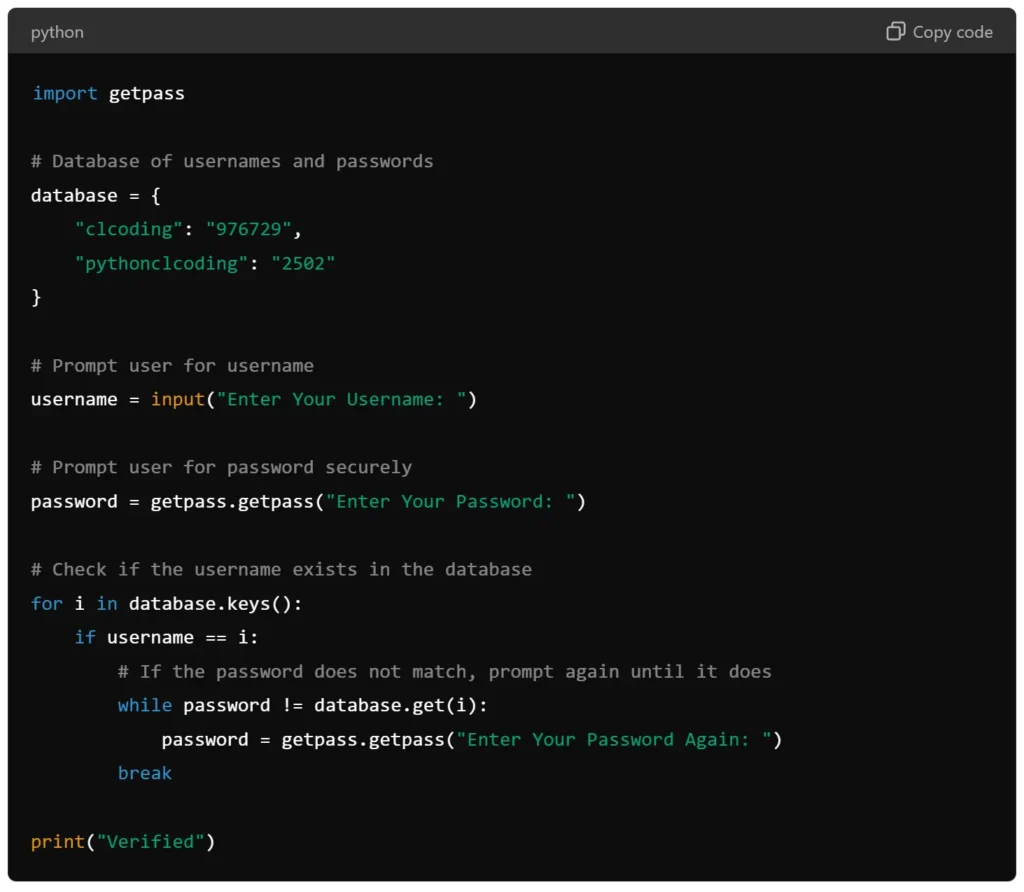
How It Works
Importing the getpass module: This module allows us to securely handle password input, preventing the password from being displayed on the screen.
Database of usernames and passwords: In this example, we use a dictionary to store usernames and their corresponding passwords. In a real-world application, passwords should be hashed and stored securely.
Prompting the user for their username and password: We use input() to get the username and getpass.getpass() to get the password.
Checking the username and password: We iterate through the database keys to check if the entered username exists. If it does, we compare the entered password with the stored password. If they don’t match, the user is prompted to enter the password again until it matches.
Verification message: Once the password matches, a verification message is printed.
Enhancing Security
The basic implementation shown above is not secure enough for real-world applications. Here are some steps to enhance security:x`
1. Hashing Passwords
Storing plain text passwords is a significant security risk. Instead, you should hash passwords before storing them. Python’s hashlib module can be used for this purpose.
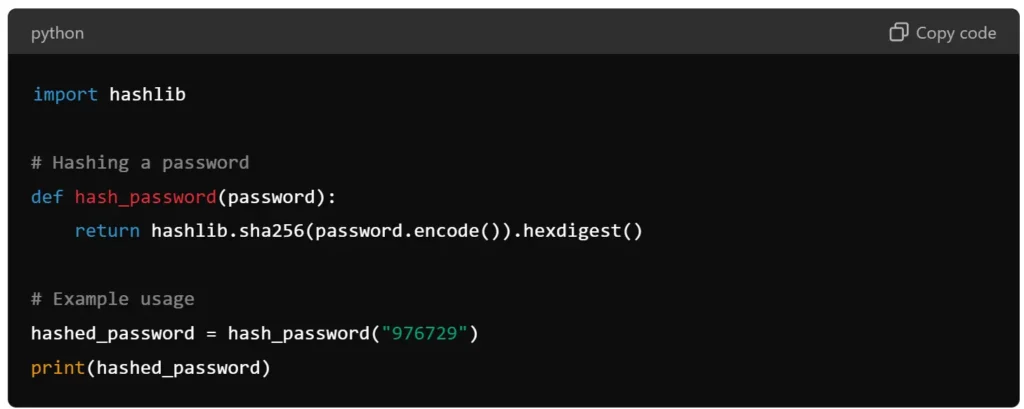
2. Salting Passwords
To further enhance security, add a unique salt to each password before hashing. This prevents attackers from using precomputed hash tables (rainbow tables) to crack passwords.
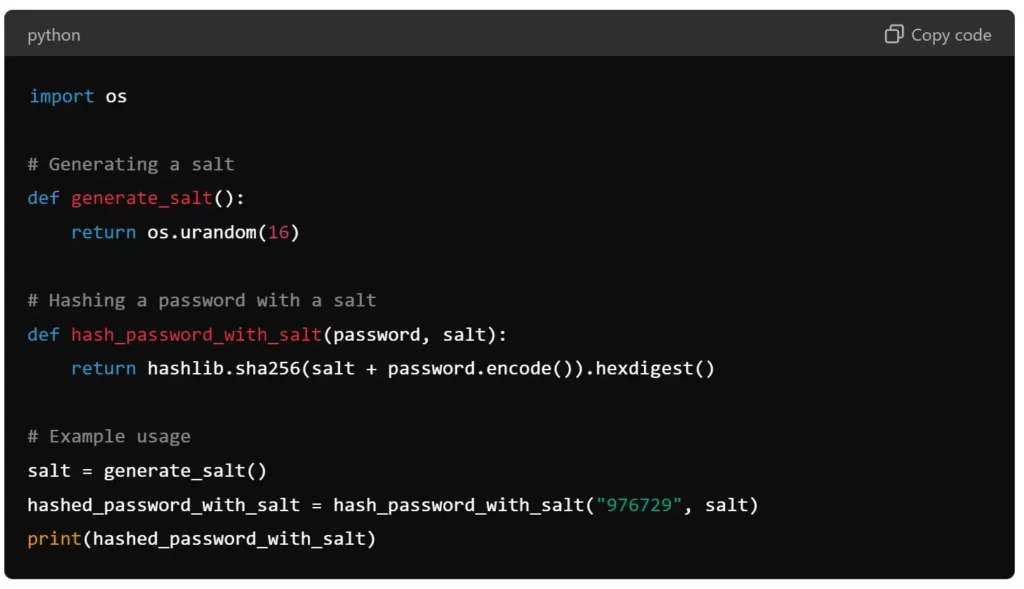
3. Using a Secure Authentication Library
For production applications, consider using a secure authentication library such as bcrypt. This library handles both hashing and salting for you and is designed to be secure against brute force attacks.
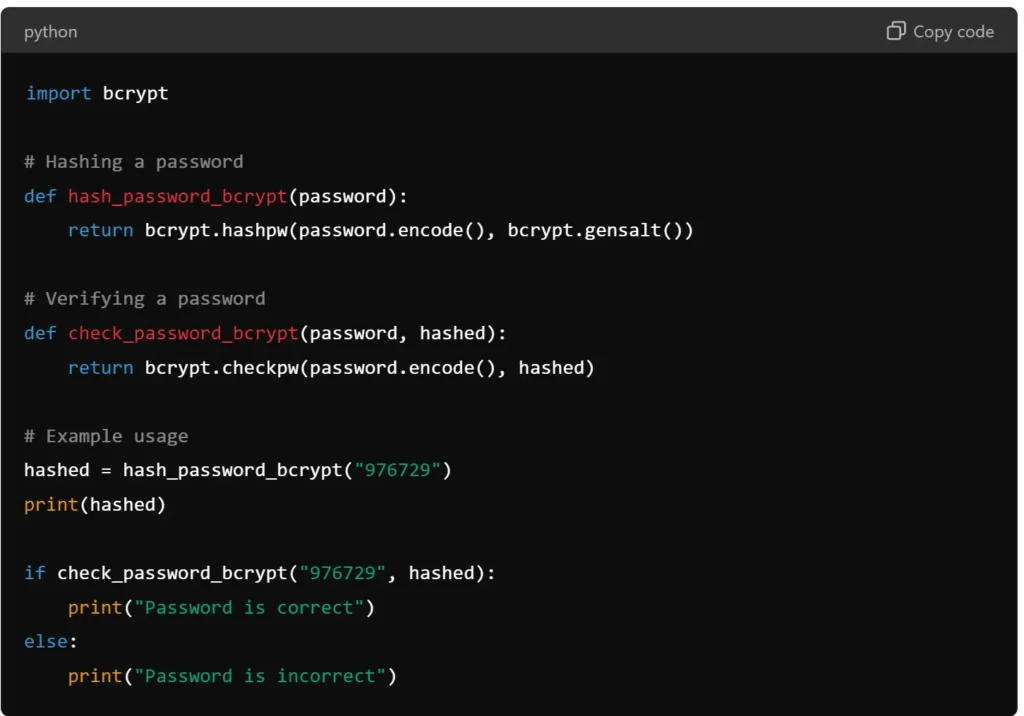
Advanced Password Authentication Example
Here’s a more advanced example incorporating password hashing and user input validation:
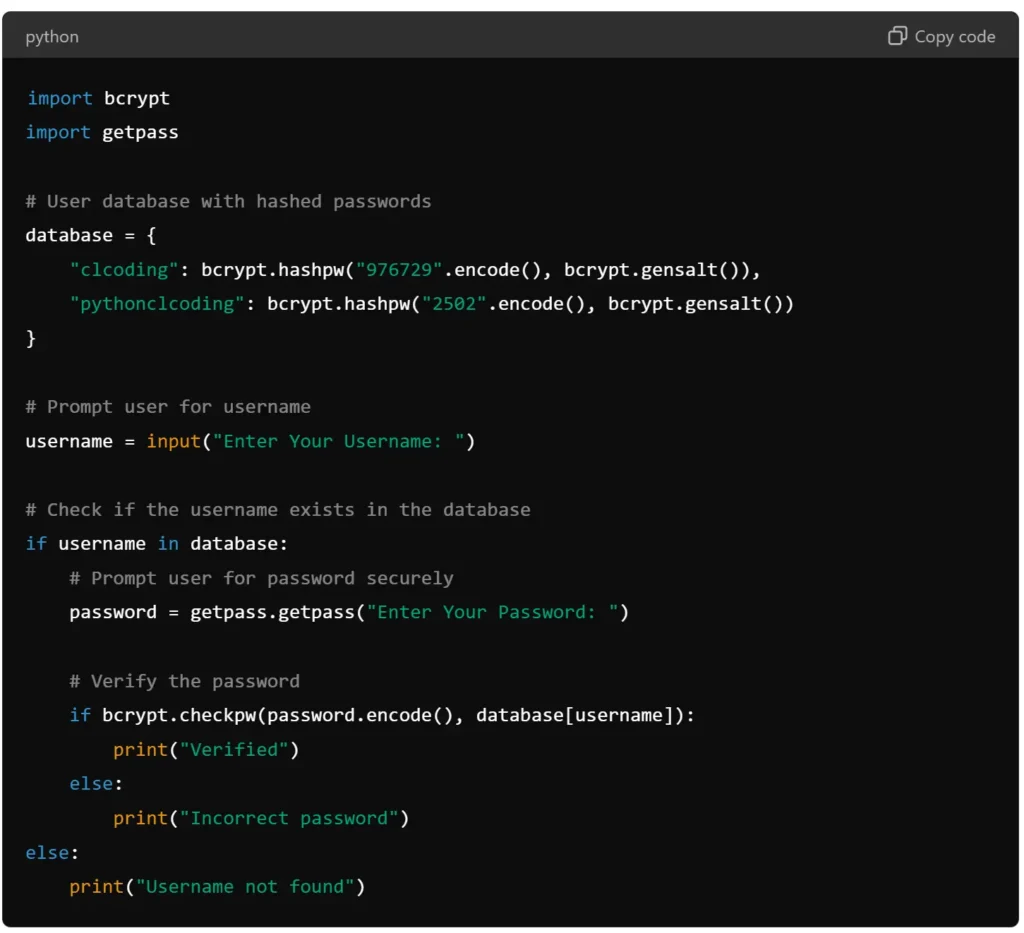
How It Works
Importing necessary modules: We import bcrypt for hashing and getpass for secure password input.
User database with hashed passwords: Passwords are hashed using bcrypt and stored in the database.
Prompting the user: We prompt the user for their username and password.
Username and password verification: We check if the username exists in the database. If it does, we use bcrypt.checkpw() to verify the entered password against the stored hashed password.
Conclusion: Password Authentication using Python
Password authentication is a crucial aspect of securing your application. By implementing the steps outlined in this guide, you can create a robust password authentication system using Python. Remember to always hash and salt passwords before storing them and consider using a secure authentication library like bcrypt for enhanced security.